File Handling in Python: Read, Write, and Manage Files with Ease
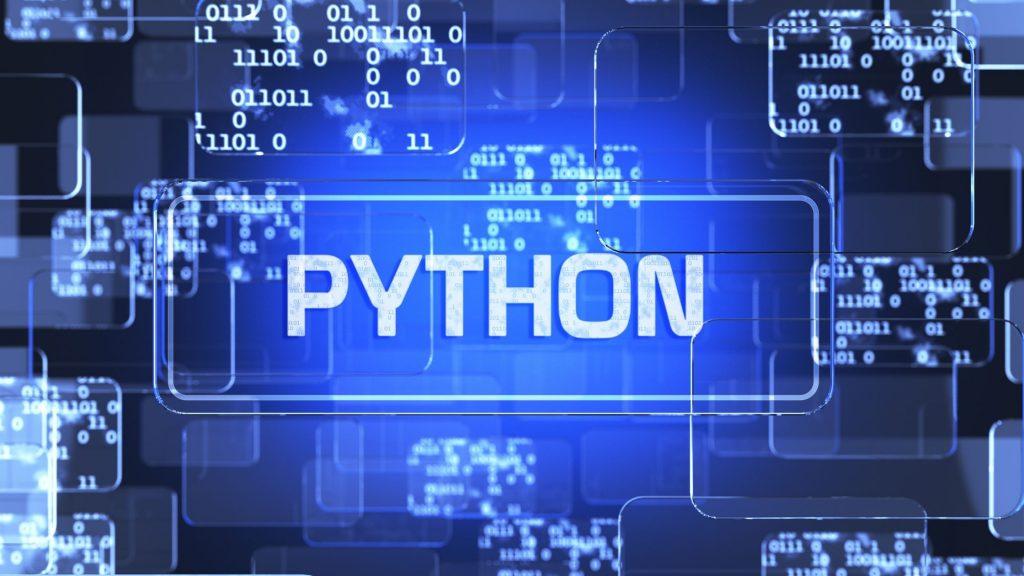
Table Of Content
- File Handling in Python: Read, Write, and Manage Files with Ease
- Opening and Closing Files
- 1. **Opening a File**
- 2. **Closing a File**
- 3. **Using `with` Statements**
- Reading Files
- 1. **Read Entire File**
- 2. **Read Line by Line**
- 3. **Read Lines into a List**
- Writing to Files
- 1. **Write to a New File**
- 2. **Append to an Existing File**
- 3. **Write Multiple Lines**
- Common File Operations
- 1. **Check if a File Exists**
- 2. **Delete a File**
- 3. **Handle Exceptions**
- Working with CSV Files
- 1. **Read CSV Files**
- 2. **Write CSV Files**
- Best Practices
- Next Steps
File Handling in Python: Read, Write, and Manage Files with Ease
Python simplifies file operations like reading, writing, and appending data. This guide covers everything you need to know to work with files efficiently using Python's built-in functions.
Opening and Closing Files
1. Opening a File
Use the open()
function to open a file. Specify the mode (read, write, append, etc.):
file = open("example.txt", "r") # Opens in read mode
Common Modes:
r
: Read (default)w
: Write (creates a new file or overwrites existing)a
: Append (adds to an existing file)b
: Binary mode (e.g.,rb
for reading binary files)
2. Closing a File
Always close files after use to free up resources:
file.close()
3. Using with
Statements
Automatically close files using with
:
with open("example.txt", "r") as file:
content = file.read()
Reading Files
1. Read Entire File
with open("example.txt", "r") as file:
content = file.read()
print(content)
2. Read Line by Line
with open("example.txt", "r") as file:
for line in file:
print(line.strip()) # Strip removes newline characters
3. Read Lines into a List
with open("example.txt", "r") as file:
lines = file.readlines()
print(lines) # Output: ["Line 1\n", "Line 2\n", ...]
Writing to Files
1. Write to a New File
with open("new_file.txt", "w") as file:
file.write("Hello, World!\n")
file.write("This is a new line.")
2. Append to an Existing File
with open("existing_file.txt", "a") as file:
file.write("\nAppended text!")
3. Write Multiple Lines
lines = ["Line 1\n", "Line 2\n", "Line 3\n"]
with open("multi_line.txt", "w") as file:
file.writelines(lines)
Common File Operations
1. Check if a File Exists
Use the os
module to verify file existence:
import os
if os.path.exists("example.txt"):
print("File exists!")
2. Delete a File
import os
os.remove("file_to_delete.txt")
3. Handle Exceptions
Use try-except
blocks to manage errors:
try:
with open("missing_file.txt", "r") as file:
print(file.read())
except FileNotFoundError:
print("File not found!")
Working with CSV Files
1. Read CSV Files
Use the csv
module for structured data:
import csv
with open("data.csv", "r") as file:
reader = csv.reader(file)
for row in reader:
print(row)
2. Write CSV Files
import csv
data = [["Name", "Age"], ["Alice", 25], ["Bob", 30]]
with open("output.csv", "w") as file:
writer = csv.writer(file)
writer.writerows(data)
Best Practices
-
Always Use
with
Statements
Ensures files are closed automatically, even if errors occur. -
Handle Exceptions
Prevent crashes due to missing files or permission issues. -
Avoid Hardcoding Paths
Use relative paths or configuration files for flexibility.
Next Steps
- Practice reading and writing text, CSV, and JSON files.
- Explore the
os
andshutil
modules for advanced file management. - Build projects like a log file analyzer or a data backup script.
Python’s file handling capabilities make it easy to work with data stored in files. Happy coding!