Python Programming
Getting Started with Python: A Beginner's Guide to Python Programming
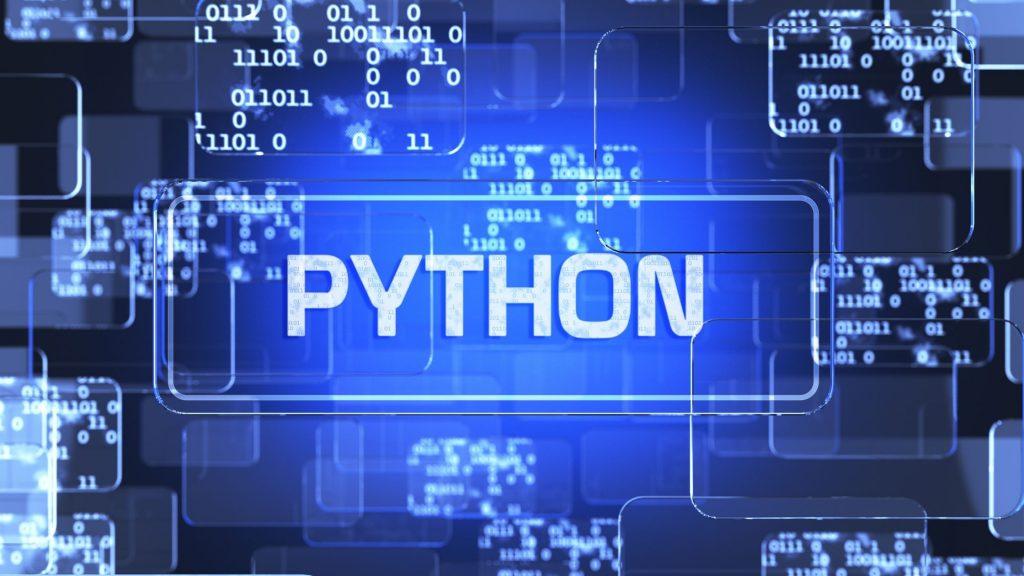
3 min read
#Python ProgrammingTable Of Content
- Getting Started with Python: A Beginner's Guide to Python Programming
- Why Learn Python?
- How to Install Python
- 1. **Download Python**
- 2. **Install Python**
- 3. **Verify Installation**
- Writing Your First Python Program
- 1. **Using the Python Interpreter**
- 2. **Creating a Python Script**
- 3. **Running the Script**
- Python Basics
- 1. **Variables and Data Types**
- 2. **Conditional Statements**
- 3. **Loops**
- 4. **Functions**
- Next Steps
Getting Started with Python: A Beginner's Guide to Python Programming
Python is one of the most popular programming languages in the world, known for its simplicity and versatility. Whether you're a complete beginner or an experienced developer exploring a new language, this guide will help you get started with Python programming.
Why Learn Python?
Python is widely used for:
- Web Development (Django, Flask)
- Data Science and Machine Learning (Pandas, NumPy, TensorFlow)
- Automation and Scripting
- Game Development (Pygame)
- Desktop Applications (Tkinter, PyQt)
Its simple syntax and readability make it an excellent choice for beginners.
How to Install Python
1. Download Python
- Visit the official Python website: python.org.
- Download the latest version of Python for your operating system (Windows, macOS, or Linux).
2. Install Python
- Run the installer and follow the instructions.
- Make sure to check the box that says "Add Python to PATH" during installation.
3. Verify Installation
- Open your terminal or command prompt and type:
python --version
- If Python is installed correctly, you'll see the version number (e.g.,
Python 3.11.2
).
Writing Your First Python Program
1. Using the Python Interpreter
- Open your terminal or command prompt and type
python
to start the Python interpreter. - Type the following code and press Enter:
print("Hello, World!")
- You should see the output:
Hello, World!
2. Creating a Python Script
- Open a text editor (e.g., Notepad, VS Code, or PyCharm).
- Write the following code:
# This is a simple Python script print("Welcome to Python Programming!")
- Save the file with a
.py
extension, e.g.,first_program.py
.
3. Running the Script
- Open your terminal or command prompt.
- Navigate to the folder where you saved the script.
- Run the script by typing:
python first_program.py
- You should see the output:
Welcome to Python Programming!
Python Basics
1. Variables and Data Types
- Python supports various data types, including integers, floats, strings, and booleans.
- Example:
name = "Alice" # String age = 25 # Integer height = 5.6 # Float is_student = True # Boolean
2. Conditional Statements
- Use
if
,elif
, andelse
to make decisions in your code. - Example:
age = 18 if age >= 18: print("You are an adult.") else: print("You are a minor.")
3. Loops
- Use
for
andwhile
loops to repeat tasks. - Example:
for i in range(5): print(f"Loop iteration: {i}")
4. Functions
-
Define reusable blocks of code using functions.
-
Example:
def greet(name): print(f"Hello, {name}!") greet("Alice")
Next Steps
Now that you've written your first Python program and learned the basics, here are some next steps:
- Explore Python libraries like NumPy and Pandas for data analysis.
- Learn about object-oriented programming (OOP) in Python.
- Build small projects like a calculator, to-do list, or a simple game.
Python is a powerful language with endless possibilities. Happy coding!