Python Syntax
Python Syntax 101: Learn the Basics of Python Programming
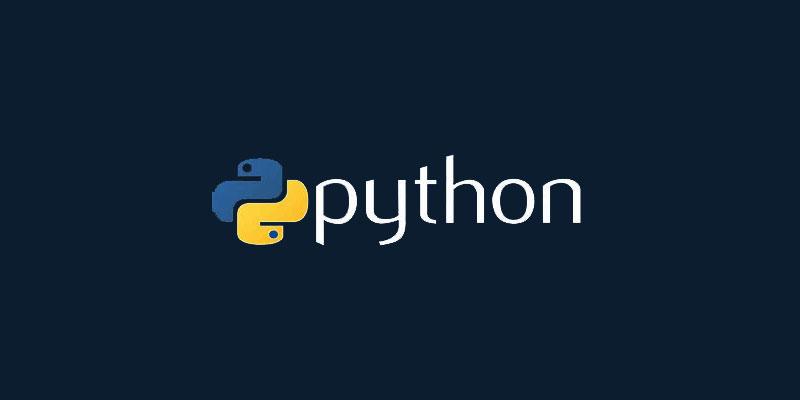
3 min read
#Python SyntaxTable Of Content
- Python Syntax 101: Learn the Basics of Python Programming
- Variables and Data Types
- 1. **Variables**
- 2. **Data Types**
- Conditional Statements
- 1. **If Statement**
- 2. **If-Else Statement**
- 3. **If-Elif-Else Statement**
- Loops
- 1. **For Loop**
- 2. **While Loop**
- Functions
- 1. **Defining Functions**
- 2. **Returning Values**
- Next Steps
Python Syntax 101: Learn the Basics of Python Programming
Python is known for its clean and readable syntax, making it an excellent choice for beginners. In this guide, we'll cover the fundamental building blocks of Python programming, including variables, data types, loops, and conditional statements.
Variables and Data Types
1. Variables
- Variables are used to store data. In Python, you don't need to declare the type of a variable explicitly.
- Example:
name = "Alice" # String age = 25 # Integer height = 5.6 # Float is_student = True # Boolean
2. Data Types
- Python supports several data types, including:
- Strings: Text data (e.g.,
"Hello, World!"
) - Integers: Whole numbers (e.g.,
42
) - Floats: Decimal numbers (e.g.,
3.14
) - Booleans: True or False values
- Strings: Text data (e.g.,
- Example:
type(name) # Output: <class 'str'> type(age) # Output: <class 'int'> type(height) # Output: <class 'float'> type(is_student) # Output: <class 'bool'>
Conditional Statements
1. If Statement
- Use
if
to execute code based on a condition. - Example:
age = 18 if age >= 18: print("You are an adult.")
2. If-Else Statement
- Use
else
to handle cases where theif
condition is false. - Example:
age = 16 if age >= 18: print("You are an adult.") else: print("You are a minor.")
3. If-Elif-Else Statement
- Use
elif
to check multiple conditions. - Example:
score = 85 if score >= 90: print("Grade: A") elif score >= 80: print("Grade: B") else: print("Grade: C")
Loops
1. For Loop
- Use
for
to iterate over a sequence (e.g., a list or range). - Example:
for i in range(5): print(f"Loop iteration: {i}")
2. While Loop
- Use
while
to repeat code as long as a condition is true. - Example:
count = 0 while count < 5: print(f"Count: {count}") count += 1
Functions
1. Defining Functions
-
Use
def
to define a function. -
Example:
def greet(name): print(f"Hello, {name}!") greet("Alice")
2. Returning Values
-
Use
return
to send a value back from a function. -
Example:
def add(a, b): return a + b result = add(3, 5) print(result) # Output: 8
Next Steps
Now that you've learned the basics of Python syntax, here are some next steps:
- Practice writing small programs using variables, loops, and functions.
- Explore Python libraries like NumPy and Pandas for advanced tasks.
- Build projects like a calculator, to-do list, or a simple game.
Python is a versatile language with endless possibilities. Happy coding!